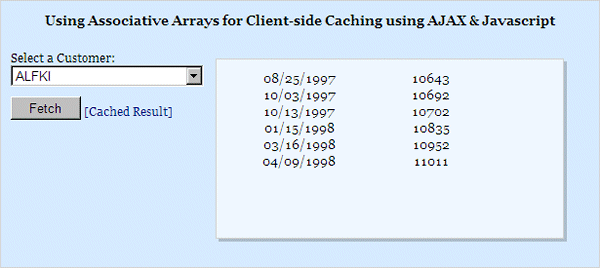
Introduction
This article shows you a useful technique to cache some of that page data on the browser in-memory. Since we use Ajax, the page is not reloaded each time. This prevents the cache from being invalidated on each round-trip. Client-side caching is sometimes required for performance reasons and also to take the load off of the DBMS. Since most modern PCs have plenty of RAM to spare, client-side caching becomes an important weapon in the modern programmer's arsenal of tricks.
With the advent of Ajax, application data can be maintained on the client without the use of ViewStates (aka hidden fields) or to a lesser degree, cookies. As any experienced developer will tell you, ViewState is a double-edged sword. Indiscriminate use of ViewStates can really have a detrimental effect on performance - what with the Viewstate payload ferried to and from the server with every postback.
The accompanying code can be extended to use record data too, via Javascript and the presentation logic can also be hived off to a Javascript routine on the client. We could even pipe XML down to the client and it could be parsed and presented through client-side script.
To keep this sample code easy to comprehend, I have rendered the tabular data from within the Web Service itself - which may not be architecturally optimal. Since this is not a treatise on design best-practices, I have taken the liberty of cutting corners here and there for the sake of brevity.
img width="36" height="42" style="float: left;" alt="Screenshot - article1.gif" src="Client-sideCaching/article1.gif" />We use JavaScript associative arrays to maintain our client-side cache. One thing to be noted about JS associative arrays is that once you associate a string key with an array, there is no way to iterate through the array using indexes. Supposing you are using it like this:
asarray["akey"] = <value>
there is no way you can revert to accessing the elements by their ordinal position like this: var anyvar = asarray[0]
. Using the code
Let us examine the critical sections of the code that are absolutely necessary for client-side caching to work. The rest of the code deals with presentation and layout and a discussion on these aspects will be skipped.For those who subscribe to the view that source-code is the ultimate documentation, you may skip this section. For the others, we'll try to separate the wheat from the chaff. Let us look at the most important pieces of code that makes client-side caching with Ajax and Javascript possible. I am using XML data for demonstration purposes and this makes the project run without having to change connection strings or even having SQL Server installed on your computer.
Step 1
Create a New Website and choose "ASP.NET AJAX-Enabled Website". If you do not have .NET 2.0 Ajax Toolkit installed, go here and download them. You cannot begin this exercise without it.Step 2
Select "Add New Item" by right-clicking on the project in Solution Explorer and choose "Web Service". Add the highlighted code to your web service class. The[ScriptService]
attribute decoration makes the web service class callable by the Ajax framework. You have to include using System.Web.Script.Services
to be able to do that. using System.Web.Script.Services;
...
[ScriptService]
public class DataAccess : System.Web.Services.WebService
{ ...
Step 3
Flesh out your web method to do something useful. Our example does this:[WebMethod]
public string FetchOrders(string key)
{
return LoadData(key);;
}
private string LoadData(string key)
{
DataSet ds = new DataSet();
ds.ReadXml(this.Context.Server.MapPath("App_Data\\northwind.xml"));
ds.Tables["Orders"].DefaultView.RowFilter = "customerid = '" + key + "'";
return RenderResultTable((ds.Tables["Orders"].DefaultView));
}
private string RenderResultTable(DataView rdr)
{
StringBuilder str = new StringBuilder();
str.AppendLine("
"); str.AppendLine(""); foreach(DataRowView drv in rdr) { str.AppendLine(" "); str.AppendFormat("", drv["OrderDate"], drv["OrderID"]); str.AppendLine(""); } str.AppendLine("
Order Date | Order ID |
{0} | {1} |
Step 4
Now, we go to the client script that does the actual caching and retrieves data by making a call to the web service. This employs rudimentary JavaScript - no surprises here.var cacheData = new Array(); // This is our cache
var currSel = null;
var seedData = "0BFA4D6B-DD18-48aa-A926-B9FD80BFA5B7";
// Prime a cache item with a unique initial value
Step 5
Add the needed JavaScript code now. We have a HTML button with the IDbtnFetch
. In the OnClick event, we trigger either an Ajax asynchronous call to the server or render from the cache.function btnFetch_onclick()
{
currSel = document.getElementById("dlCustomers").value;
var status = document.getElementById("divStatus");
//var svc = new DataAccess();
if(cacheData[currSel]==null)
{
DataAccess.FetchOrders(currSel,OnCompleteCallback,OnErrorCallback,
OnTimeOutCallback);
cacheData[currSel] = seedData;
status.innerHTML = "[Live Result]";
}
else
{
status.innerHTML = "[Cached Result]";
var cacheobject = FetchDataFromCache(currSel);
RenderData(cacheobject);
}
document.getElementById("dlCustomers").focus();
}
0 comments:
Post a Comment